Introducing 'tremendousr'
By Jonathan Trattner in R Packages
September 30, 2021
Introduction
I was recently asked to explore alternatives for Amazon’s Mechanical Turk and Prolific with the goal of recruiting people from targeted populations to participate in behavioral tasks. As a dedicated #rstats user, I’ve been developing these tasks using shiny and shinysurveys. One challenge was how to compensate people for their time. That’s where Tremendous comes in. Tremendous is a platform that “empowers companies to buy, track, and manage digital and physical payments.” They provide an API that allows you to send and manage rewards dynamically, which is exactly what I needed.
There was not an API wrapper for R yet, so I wrote tremendousr to provide a (slightly-opinionated) interface for Tremendous’s API. In this post, I’ll briefly walk-through how to get started sending rewards. Much of the information is available in the README, and I cover additional examples certain API endpoints, in the “Getting Started” vignette.
A note on package conventions: All functions begin with the prefix
trem_
, followed by categories such asclient
or action verbs such asget
orpost
. This allows you to take advantage of RStudio’s auto-completion feature and quickly accomplish your goals.
How do you Install ‘tremendousr’?
You can install tremendousr via CRAN or GitHub and load it as follows:
# Install released version from CRAN
install.packages("tremendousr")
# Or, install the development version from GitHub
remotes::install_github("jdtrat/tremendousr")
# Load package
library(tremendousr)
Sending your First Payment
Background
Tremendous provides two environments for their platform:
‘Sandbox’ environment, a “free and fully-featured environment for application development and testing.”
‘Production’ environment, where real payments can be sent.
Tremendous API users typically develop their applications against the sandbox environment, and then switch their credentials to production when they are ready to go live.
To begin, you must create a Tremendous account. For the sandbox environment, you can sign up or log-in here and generate an API key by navigating to Team Settings > Developers and clicking on Add API key on the top right. You can follow the official documentation here.
With an API key, you can create a Tremendous Client in R and begin interacting with Tremendous.
Create a Tremendous Client
In order to send a payment, or access other features of Tremendous, you must use a Tremendous Client, which you can create with the function trem_client_new()
. This is a convenient way to bundle your authentication (API) credentials and ensure you are using the proper environment (sandbox or production) for your calls. Conveniently, Tremendous prefixes API keys for each environment to differentiate them. If you’re using the sandbox environment, your API key would begin with TEST_
; the production environment key will begin with PROD_
.
test_client <- trem_client_new(api_key = "TEST_YOUR-KEY-HERE",
sandbox = TRUE)
# Print Tremendous API Client
test_client
#> <tremendousClient>
#> API key: <private>
#> API Environment: Sandbox
Sending a Reward
In Tremendous, rewards start out as orders which contain information about the recipient, reward amount and type, funding source, delivery method, and a payment description id (what they refer to as an ‘external id’). Orders can be fulfilled either immediately or upon approval by your Tremendous Account administrator. To turn on approvals, you can login to your Tremendous account and navigate to Team Settings > Approvals and click Order Approvals: OFF to toggle it on.
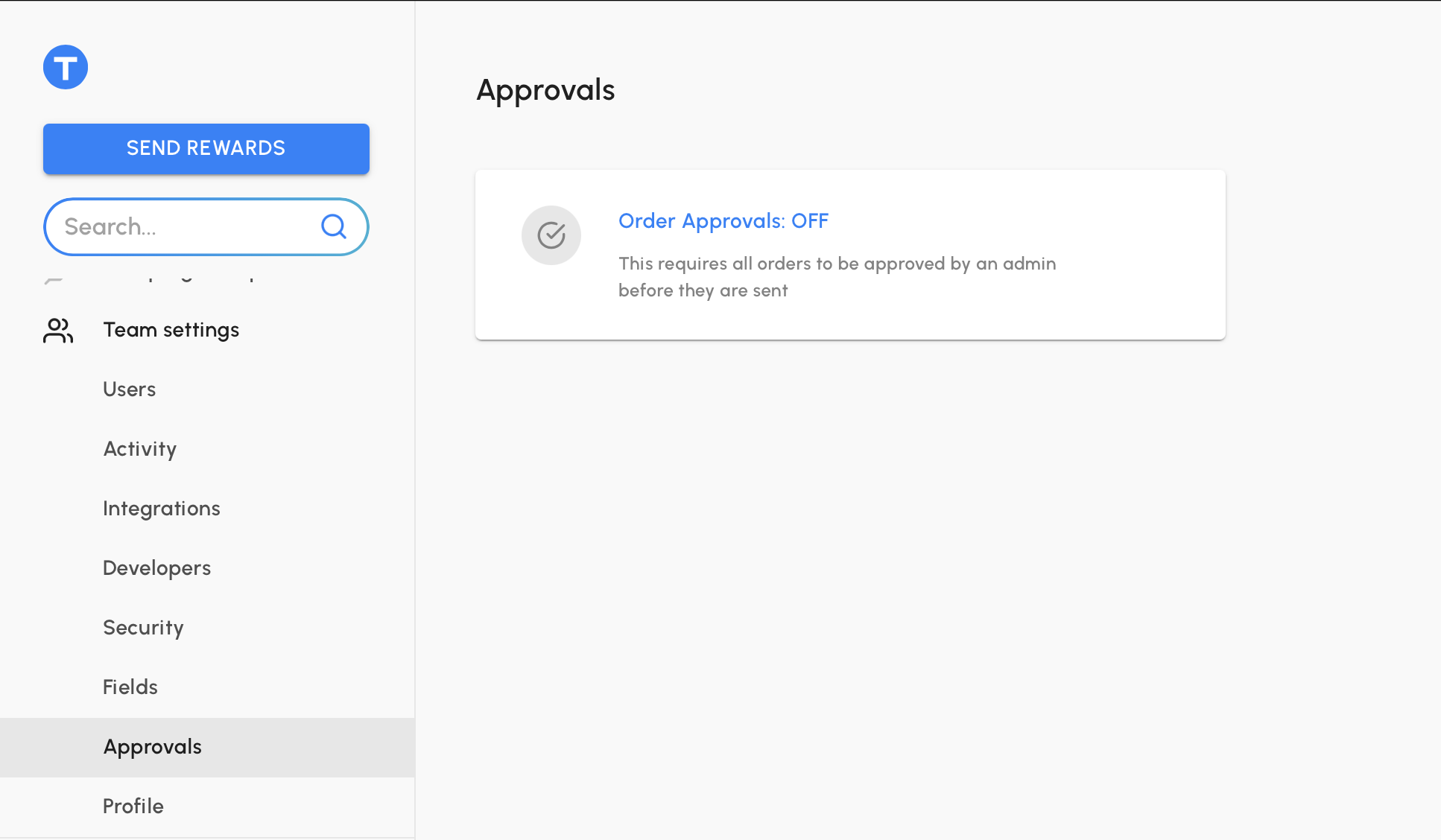
A screenshot from the Tremendous Web Portal showing the ‘Order Appovals’ setting.
In general, when working in the ‘Sandbox’ environment, I would not require order approvals. I think this helps you refine payment methods, reward options, etc. more quickly.1 To take my own advice, in this post, I’ll keep orders approval off. Either way, though, to create an order and have the reward send (immediately or upon approval), you should use the function trem_send_reward()
as follows:
trem_send_reward(client = test_client,
name = "first last",
email = "email@website.com",
reward_amount = 10,
currency_code = "USD",
delivery_method = "EMAIL",
payment_description_id = "payment-from-intro-to-tremendousr",
funding_source_id = "your-funding-id-from-tremendous",
reward_types = "Q24BD9EZ332JT", # ID for virtual visa gift card
parse = TRUE # Return a parsed API response
)
Under the hood, this function performs a POST request for creating an order using Tremendous.2 For detailed documentation on each field, I encourage you to check out the function’s help documentation (?trem_send_reward
) or the official Tremendous documentation.
In sending this to myself, I get an email with a link to a redeem a Visa gift card:
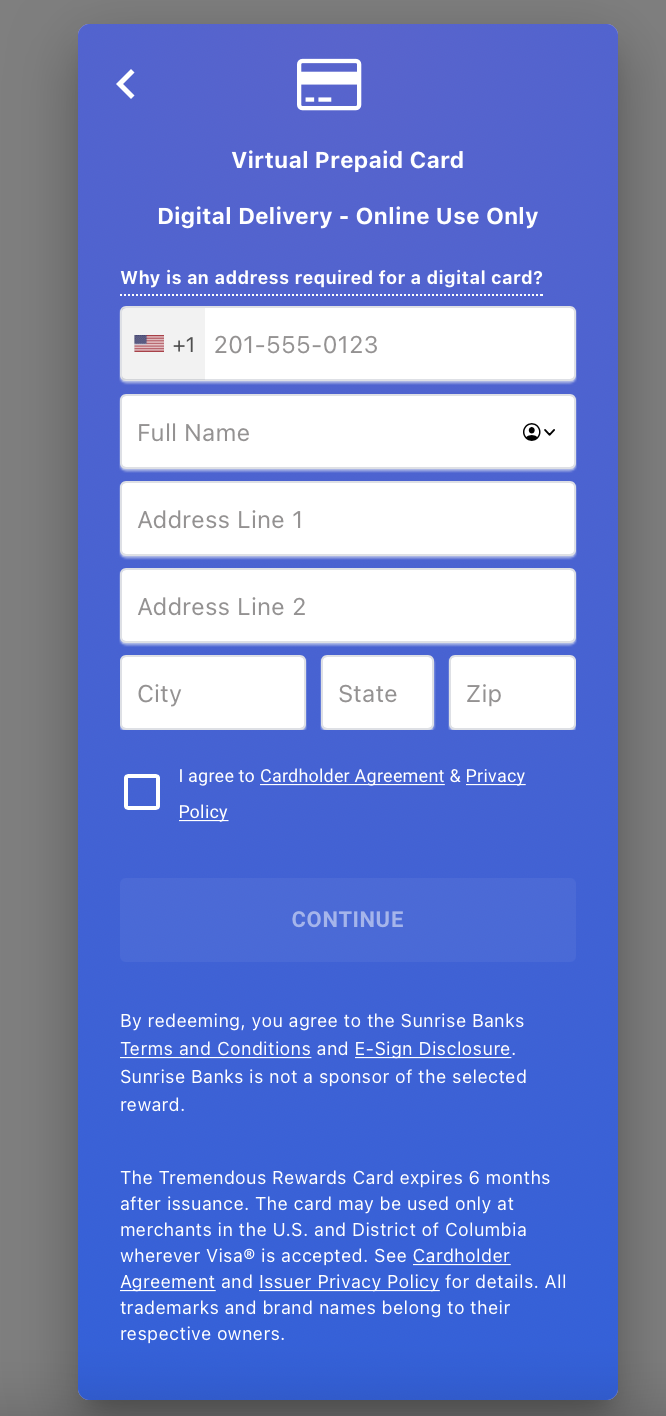
Preview of what redeeming a Virtual Visa Gift Card looks like. A blue box with a title “Virtual prepaid card” followed by contact information fields and terms of service information.
Thoughts, Tips, and Tricks
I have really enjoyed working with the Tremendous API and found it quite intuitive. As such, for many of the endpoints, I have opted (for now, at least) to not create wrapper functions since they will not save a lot, if any, time. For example, to list your reward history you would call:
trem_get(test_client, "rewards")
To access a specific reward’s information, you would simply append the reward ID:
trem_get(test_client, "rewards/REWARD_ID")
In a future release of tremendousr, perhaps I will wrap this in a utility function such as trem_get_reward()
, but I think this is user-friendly and encourages checking the API documentation 🤓
Setting your API key
It is best practice to never include your API key within your R script in the event you inadvertently share it. To help with this, I include a utility function, trem_set_api_key()
, which can (and should) be used as follows.
trem_set_api_key("TEST_YOUR-KEY-HERE")
#> • You may wish to add your Tremendous Test API key to your '.Renviron' file:
#> TREMENDOUS_TEST_KEY=TEST_YOUR-KEY-HERE
#> [Copied to clipboard]
#> • To edit your '.Renviron' file:
#> - Check that usethis is installed.
#> - Call `usethis::edit_r_environ()`.
#> - Check that '.Renviron' ends with a new line.
test_client <- trem_client_new(api_key = NULL, # Accesses environmental variable
sandbox = TRUE)
Setting a Funding ID
In the sandbox environment, from what I understand, there is only one funding source (with a $5,000 limit that resets daily). Using the trem_get()
function, you can easily define a variable for using trem_send_reward()
as follows:
funding_id <- trem_get(test_client, "funding_sources")$funding_sources$id
Finding Payment Methods
Tremendous offers support for over 600 reward types, including Virtual Visa, Starbucks, and Amazon gift cards, as well as ACH transfers. When sending a reward, you can define as many reward types, using a character vector of the ID, which will allow them to choose what they prefer.
I think the easiest way of finding reward types is by browsing their online catalog. Using the name, you can then access their IDs as shown below. For this example, I’ll get the IDs for ‘Best Buy’, ‘Virtual Visa’, and ‘Target’ gift cards, making sure the currency is US dollars. I’m going to use the package dplyr for easy data manipulation and to show how you can use the pipe3 to pass the Tremendous API client around.
library(dplyr)
library(magrittr)
# Save the current product listing as 'prods'
prods <- test_client %>%
trem_get("products")
# Access the 'products' data frame of prods and filter it to desired reward types, assigning the IDs as 'reward_ids'.
reward_ids <- prods$products %>%
# Filter so the name is either "Virtual Visa", "Target", or "Best Buy" AND the currency code is "USD"
filter(name %in% c("Virtual Visa", "Target", "Best Buy") & currency_codes == "USD") %>%
# Grab the IDs
pull(id)
I did not include the product data in the package because – from my understanding – the IDs, and available options, are subject to change.
Have a ✨Tremendous✨ Day!
Thanks for reading my blog post! I hope you find use for tremendousr (and, of course, Tremendous). If you have any feedback, I’d love for you to file an issue. For more of my work, check out my GitHub! If you want to chat about anything (including neuroscience, #rstats, piano, or my kitten), DM me on Twitter! Lastly, if you need help with an #rstats or shiny project, I’m available for consulting – just send me an email!
When you are ready to send actual rewards within the ‘Production’ environment, you may wish to have this feature enabled, especially if you will be automating reward delivery as an extra layer of protection.↩︎
The help documentation for
trem_post()
actually shows an example of sending a reward manually. I find this wrapper function easier to work with, though, than nested lists 😅↩︎